The Language Reference - Functions
Overview
The Arduino language is based on C/C++ and supports standard functions as well as Arduino-specific ones.
I/O
Digital I/O
pinMode(pin, mode)
Configures the specified pin to behave either as an INPUT
or an OUTPUT
.
It is possible to enable the internal pull-up resistors with the mode INPUT_PULLUP
. Additionally, the INPUT
mode explicitly disables the internal pull-up.
Parameters:
pin
: the Arduino pin number to set the mode of.mode
:INPUT
,OUTPUT
, orINPUT_PULLUP
.
Returns: Nothing.
digitalRead(pin)
Reads the value from a specified digital pin, either HIGH
or LOW
.
Parameters
pin
: the Arduino pin number you want to read.
Returns: The boolean state of the read pin as HIGH
or LOW
.
digitalWrite(pin, value)
Write a HIGH
or a LOW
value to a digital pin.
- If the pin has been configured as an
OUTPUT
withpinMode()
, its voltage will be set to the corresponding value: 5V (or 3.3V on 3.3V boards) for HIGH and 0V (ground) for LOW. - If the pin is configured as an
INPUT
,digitalWrite()
will enable (HIGH) or disable (LOW) the internal pull-up on the input pin. It is recommended to set the pinMode() to INPUT_PULLUP to enable the internal pull-up resistor. - If you do not set the pin as an OUTPUT, and connect an LED to it, when calling
digitalWrite(pin, HIGH)
, the LED may appear dim. Without explicitly settingpinMode()
,digitalWrite()
will have enabled the internal pull-up resistor, which acts like a large current-limiting resistor.
Parameters:
pin
: the Arduino pin number to be controlled.value
:HIGH
orLOW
Returns: Nothing
Analog I/O
analogRead(pin)
Reads the value from the specified analog pin.
Parameters:
pin
: the name of the analog input pin to read from.
Returns: The analog reading on the pin. Although it is limited to the resolution of the analog to digital converter (0-1023 for 10 bits or 0-4095 for 12 bits). Data type: int.
analogWrite(pin, value)
Writes an analog value (PWM wave) to a pin. Can be used to light a LED at varying brightnesses or drive a motor at various speeds. After a call to analogWrite()
, the pin will generate a steady rectangular wave of the specified duty cycle until the next call to analogWrite()
(or a call to digitalRead()
or digitalWrite()
) on the same pin.
Parameters:
pin
: the Arduino pin to write to. Allowed data types: intvalue
: the duty cycle: between 0 (always off) and 255 (always on). Allowed data types: int
Returns: Nothing
analogReadResolution(bits)
Sets the size (in bits) of the value returned by analogRead()
. It defaults to 10 bits (returns values between 0-1023) for backward compatibility with AVR based boards.
You can set this between 1 and 32. You can set resolutions higher than the supported 12 or 16 bits, but values returned by analogRead() will suffer approximation. If you set the analogReadResolution() value to a value higher than your board’s capabilities, the Arduino board will only report back at its highest resolution, padding the extra bits with zeros.
Parameters:
bits
: determines the resolution (in bits) of the value returned by the analogRead() function.
Returns : Nothing
Parameters:
type
: which type of reference to use. The options differ by types of boards.
Returns: Nothing
analogWriteResolution(bits)
Sets the resolution of the analogWrite()
function. It defaults to 8
bits (values between 0-255) for backward compatibility with AVR based boards.
Parameters:
bits
: determines the resolution (in bits) of the values used in the analogWrite() function. The value can range from 1 to 32.
Returns: Nothing
analogReference(type)
Configures the reference voltage used for analog input (i.e. the value used as the top of the input range).
After changing the analog reference, the first few readings from analogRead()
may not be accurate.
Don’t use anything less than 0V or more than 5V for external reference voltage on the AREF pin. If you’re using an external reference on the AREF pin, you must set the analog reference to EXTERNAL before calling analogRead().
Advanced I/O
tone(pin, frequency[, duration])
Generates a square wave of the specified frequency (and 50% duty cycle) on a pin.
Parameters:
pin
: the Arduino pin on which to generate the tone.frequency
: the frequency of the tone in hertz. Allowed data types: unsigned int.duration
: the duration of the tone in milliseconds (optional). Allowed data types: unsigned long.
Returns: Nothing
noTone(pin)
Stops the generation of a square wave triggered by tone(). Has no effect if no tone is being generated.
Parameters:
pin
: the Arduino pin on which to stop generating the tone
Returns: Nothing
pulseIn(pin, value[, timeout])
Reads a pulse (either HIGH
or LOW
) on a pin.
Parameters
pin
: the number of the Arduino pin on which you want to read the pulse. Allowed data types: int.value
: type of pulse to read: eitherHIGH
orLOW
. Allowed data types: int.timeout
(optional): the number of microseconds to wait for the pulse to start; default is one second. Allowed data types: unsigned long.
Returns: The length of the pulse (in microseconds) or 0 if no pulse started before the timeout. Data type: unsigned long.
pulseInLong(pin, value[, timeout])
Reads a pulse (either HIGH
or LOW
) on a pin. pulseInLong()
is an alternative to pulseIn()
which is better at handling long pulse and interrupt affected scenarios.
Parameters:
pin
: the number of the Arduino pin on which you want to read the pulse. Allowed data types: int.value
: type of pulse to read: either HIGH or LOW. Allowed data types: int.timeout
(optional): the number of microseconds to wait for the pulse to start; default is one second. Allowed data types: unsigned long.
Returns: The length of the pulse (in microseconds) or 0 if no pulse started before the timeout. Data type: unsigned long.
shiftIn(dataPin, clockPin, bitOrder)
Shifts in a byte of data one bit at a time. Starts from either the most (i.e. the leftmost) or least (rightmost) significant bit. For each bit, the clock pin is pulled high, the next bit is read from the data line, and then the clock pin is taken low.
Note: This is a software implementation; Arduino also provides an SPI library that uses the hardware implementation, which is faster but only works on specific pins.
Parameters
dataPin
: the pin on which to input each bit. Allowed data types: int.clockPin
: the pin to toggle to signal a read from dataPin.bitOrder
: which order to shift in the bits; eitherMSBFIRST
orLSBFIRST
. (Most Significant Bit First, or, Least Significant Bit First).
Returns: The value read. Data type: byte.
shiftOut(dataPin, clockPin, bitOrder, value)
Shifts out a byte of data one bit at a time. Starts from either the most (i.e. the leftmost) or least (rightmost) significant bit. Each bit is written in turn to a data pin, after which a clock pin is pulsed (taken high, then low) to indicate that the bit is available.
Note: This is a software implementation; Arduino also provides an SPI library that uses the hardware implementation, which is faster but only works on specific pins.
Parameters:
dataPin
: the pin on which to output each bit. Allowed data types: int.clockPin
: the pin to toggle once the dataPin has been set to the correct value. Allowed data types: int.bitOrder
: which order to shift out the bits; eitherMSBFIRST
orLSBFIRST
. (Most Significant Bit First, or, Least Significant Bit First).value
: the data to shift out. Allowed data types: byte.
Returns: Nothing
Interrupts
interrupts()
Re-enables interrupts (after they’ve been disabled by noInterrupts()
) Interrupts allow certain important tasks to happen in the background and are enabled by default. Some functions will not work while interrupts are disabled, and incoming communication may be ignored. Interrupts can slightly disrupt the timing of code, however, and may be disabled for particularly critical sections of code.
noInterrupts()
Disables interrupts (you can re-enable them with interrupts()). Interrupts allow certain important tasks to happen in the background and are enabled by default. Some functions will not work while interrupts are disabled, and incoming communication may be ignored. Interrupts can slightly disrupt the timing of code, however, and may be disabled for particularly critical sections of code.
attachInterrupt(interrupt, ISR, mode)
Interrupts help make things happen automatically in microcontroller programs and can help solve timing problems. Good tasks for using an interrupt may include reading a rotary encoder, or monitoring user input.
Parameters:
interrupt
: the number of the interrupt. Allowed data types: int.pin
: the Arduino pin number.ISR
: the ISR to call when the interrupt occurs; this function must take no parameters and return nothing. This function is sometimes referred to as an interrupt service routine.mode
: defines when the interrupt should be triggered. Four constants are predefined as valid values:LOW
to trigger the interrupt whenever the pin is low,CHANGE
to trigger the interrupt whenever the pin changes valueRISING
to trigger when the pin goes from low to high,FALLING
for when the pin goes from high to low.
Returns: Nothing
ISRs are special kinds of functions that have unique limitations not shared by most other functions. An ISR cannot have any parameters and it should not return anything.
Generally, an ISR should be as short and fast as possible. If your sketch uses multiple ISRs, only one can run at a time; Other interrupts will be executed after the current one finishes, in an order that depends on their priority. millis() relies on interrupts to count, so it will never increment inside an ISR. Since delay() requires interrupts to work, it will not function if called inside an ISR. micros() works initially but starts behaving erratically after 1-2 ms. delayMicroseconds() does not use a counter, so it will work as usual.
Typically, global variables are used to pass data between an interrupt service routine (ISR) and the main program. To make sure variables shared between an ISR and the main program are updated correctly, declare them as volatile.
For more information on interrupts, see Nick Gammon’s notes.
detachInterrupt(interrupt)
Turns off a given interrupt that was attached previously.
Parameters:
interrupt
: the number of the interrupt to disable (see attachInterrupt() for more details)
Returns: Nothing
digitalPinToInterrupt(pin)
To verify if a given pin can be used an interrupt.
A full list of supported interrupt pins on all boards can be found here.
Parameters
pin
: the pin we want to use for an interrupt.
Returns:
- If the pin is available for interrupt, it will return the given pin (e.g. 2).
- If the pin is not available for interrupt, it will return
-1
.
Time
delay(ms)
Pauses the program for the amount of time (in milliseconds).
Parameters:
ms
: the number of milliseconds to pause. Allowed data types: unsigned long.
Returns: Nothing
delayMicroseconds(us)
Pauses the program for the amount of time (in microseconds).
Parameters
us
: the number of microseconds to pause. Allowed data types: unsigned int.
Returns: Nothing
micros()
Returns the number of microseconds since the Arduino board began running the current program. This number will overflow (go back to zero), after approximately 70 minutes.
millis()
Returns the number of milliseconds passed since the Arduino board began running the current program. This number will overflow (go back to zero), after approximately 50 days.
Characters, Bytes and Bits
Characters
- isAlpha(thisChar): analyze if a char is alpha (that is a letter).
- isAlphaNumeric(thisChar): analyze if a char is alphanumeric (that is a letter or a number).
- isAscii(thisChar): analyze if a char is ASCII.
- isControl(thisChar): analyze if a char is a control character.
- isDigit(thisChar): analyze if a char is a digit (that is a number).
- isGraph(thisChar): analyze if a char is printable with some content (space is printable but has no content).
- isHexadecimalDigit(thisChar): analyze if a char is a hexadecimal digit (A-F, 0-9).
- isLowerCase(thisChar): analyze if a char is lower case (that is a letter in lower case).
- isPrintable(thisChar): analyze if a char is printable (that is any character that produces an output, even a blank space).
- isPunct(thisChar): analyze if a char is punctuation (that is a comma, a semicolon, an exclamation mark and so on).
- isSpace(thisChar): analyze if a char is a white-space character.
- isUpperCase(thisChar): analyze if a char is upper case (that is, a letter in upper case).
- isWhitespace(thisChar): analyze if a char is a space character.
Bytes
- highByte(x): Extracts the high-order (leftmost) byte of a word (or the second lowest byte of a larger data type).
- lowByte(x): Extracts the low-order (rightmost) byte of a variable (e.g. a word).
Bits
- bit(n): Computes the value of the specified bit (bit 0 is 1, bit 1 is 2, bit 2 is 4, etc.).
- bitClear(x, n): Clears (writes a 0 to) a bit of a numeric variable.
- bitRead(x, n): Reads a bit of a variable, e.g.
bool
,int
. Note thatfloat
&double
are not supported. - bitSet(x, n): Sets (writes a 1 to) a bit of a numeric variable.
- bitWrite(x, n, b): Writes to a bit of a variable, e.g.
bool
,int
,long
. Note thatfloat
&double
are not supported.
Math
- abs(x): calculates the absolute value of a given number.
- constrain(x, a, b): constraints a number to be within a range.
- map(value, fromLow, fromHigh, toLow, toHigh): re-maps a number from one range to another.
- max(x, y): compare two numbers and find the larger.
- min(x, y): compare two numbers and find the smaller.
- pow(base, exponent): raise a number to a given power.
- sq(x): compute the square of a number.
- sqrt(x): find the square root of a given number.
- cos(rad): calculate the cosine of a given angle in radians. The result will be between -1 and 1.
- sin(rad): calculate the sine of a given angle in radians.The result will be between -1 and 1.
- tan(rad): calculate the tangent of a given angle in radians. The result will be between negative infinity and infinity.
- random(max) / random(min, max): generates pseudo-random numbers.
- randomSeed(seed): initializes the pseudo-random number generator, causing it to start at an arbitrary point in its random sequence.
Communication
SPI
This library allows you to communicate with SPI devices, with the Arduino board as the controller device. This library is bundled with every Arduino platform (avr, megaavr, mbed, samd, sam, arc32), so you do not need to install the library separately.
Functions
- SPISettings
- begin()
- beginTransaction()
- endTransaction()
- end()
- setBitOrder()
- setClockDivider()
- setDataMode()
- transfer()
- usingInterrupt()
The Print class is an abstract base class that provides a common interface for printing data to different output devices. It defines several methods that allow printing data in different formats.
Functions
Serial
Used for communication between the Arduino board and a computer or other devices.
Functions
- if(Serial)
- available()
- availableForWrite()
- begin()
- end()
- find()
- findUntil()
- flush()
- parseFloat()
- parseInt()
- peek()
- print()
- println()
- read()
- readBytes()
- readBytesUntil()
- readString()
- readStringUntil()
- setTimeout()
- write()
- serialEvent()
Stream
Stream is the base class for character and binary based streams. It is not called directly, but invoked whenever you use a function that relies on it.
Functions
- available()
- read()
- flush()
- find()
- findUntil()
- peek()
- readBytes()
- readBytesUntil()
- readString()
- readStringUntil()
- parseInt()
- parseFloat()
- setTimeout()
Wire
This library allows you to communicate with I2C devices, a feature that is present on all Arduino boards.
Functions
- begin()
- end()
- requestFrom()
- beginTransmission()
- endTransmission()
- write()
- available()
- read()
- setClock()
- onReceive()
- onRequest()
- setWireTimeout()
- clearWireTimeoutFlag()
- getWireTimeoutFlag()
External Devices
Keyboard
The keyboard functions enable 32u4 or SAMD micro based boards to send keystrokes to an attached computer through their micro’s native USB port.
Not every possible ASCII character, particularly the non-printing ones, can be sent with the Keyboard library.
Functions
- Keyboard.begin()
- Keyboard.end()
- Keyboard.press()
- Keyboard.print()
- Keyboard.println()
- Keyboard.release()
- Keyboard.releaseAll()
- Keyboard.write()
Mouse
The mouse functions enable 32u4 or SAMD micro based boards to control cursor movement on a connected computer through their micro’s native USB port. When updating the cursor position, it is always relative to the cursor’s previous location.
Functions
Wi-Fi
The Wi-Fi API originates from the Ethernet library, and is broken out to several libraries, depending on what board and architecture used.
The API listed in the language reference is a common API that is written to accomodate all generic Wi-Fi related functions across all Wi-Fi compatible Arduino boards.
WiFi Network
The WiFi
class contains Wi-Fi specific functions such as initializing the network settings as well as connecting to & disconnecting from a network.
WiFi.begin()
: Initializes the WiFi library’s network settings and provides the current status.WiFi.disconnect()
: Disconnects the WiFi shield from the current network.WiFi.config()
: Configures a static IP address as well as change the DNS, gateway, and subnet addresses on the WiFi shield.WiFi.setDNS()
: Configures the DNS (Domain Name System) server.WiFi.SSID()
: Gets the SSID of the current network.WiFi.BSSID()
: Gets the MAC address of the router you are connected to.WiFi.RSSI()
: Gets the signal strength of the connection to the router.WiFi.encryptionType()
: Gets the encryption type of the current network.WiFi.scanNetworks()
: Scans for available WiFi networks and returns the discovered number.WiFi.status()
: Returns the connection status.WiFi.getSocket()
: Gets the first socket available.WiFi.macAddress()
: Gets the MAC Address of your WiFi shield.
IPAddress
The IPAddress
class contains methods to access the local IP address, gateway IP address and subnet mask.
IPAddress.localIP()
: Gets the WiFi shield’s IP address.IPAddress.subnetMask()
: Gets the WiFi shield’s subnet mask.IPAddress.gatewayIP()
: Gets the WiFi shield’s gateway IP address.
WiFiClient
The WiFiClient
class is used to connect, send and receive data to and from servers.
client.connected()
: Whether or not the client is connected.client.connect()
: Connect to the IP address and port.client.write()
: Write data to the server the client is connected to.client.print()
: Print data to the server that a client is connected to.client.println()
: Print data, followed by a carriage return and newline, to the server a client is connected to.client.available()
: Returns the number of bytes available for reading (that is, the amount of data that has been written to the client by the server it is connected to).client.read()
: Read the next byte received from the server the client is connected to (after the last call to read()).client.flush()
: Discard any bytes that have been written to the client but not yet read.client.stop()
: Disconnect from the server.
WiFiServer
The WiFiServer
is used for server based calls, such as creating server that listens to a specific port, or writing data to connected clients.
server.begin()
: Tells the server to begin listening for incoming connections.server.available()
: Gets a client that is connected to the server and has data available for reading.server.write()
: Write data to all the clients connected to a server.server.print()
: Print data to all the clients connected to a server. Prints numbers as a sequence of digits, each an ASCII character (e.g. the number 123 is sent as the three characters ‘1’, ‘2’, ‘3’).server.println()
: Prints data, followed by a newline, to all the clients connected to a server.
WiFiUDP
The WiFiUDP
class is used send and receive UDP messages over Wi-Fi.
WiFiUDP.begin()
: Initializes the WiFi UDP library and network settings. Starts WiFiUDP socket, listening at local port.WiFiUDP.available()
: Get the number of bytes (characters) available for reading from the buffer.WiFiUDP.beginPacket()
: Starts a connection to write UDP data to the remote connection.WiFiUDP.endPacket()
: Called after writing UDP data to the remote connection. It finishes off the packet and send it.WiFiUDP.write()
: Writes UDP data to the remote connection. Must be wrapped betweenbeginPacket()
andendPacket()
.beginPacket()
initializes the packet of data, it is not sent untilendPacket()
is called.WiFiUDP.parsePacket()
: It starts processing the next available incoming packet, checks for the presence of a UDP packet, and reports the size. parsePacket() must be called before reading the buffer withWiFiUDP.read()
.WiFiUDP.peek()
: Read a byte from the file without advancing to the next one. That is, successive calls topeek()
will return the same value, as will the next call toread()
.WiFiUDP.read()
: Reads UDP data from the specified buffer. If no arguments are given, it will return the next character in the buffer.WiFiUDP.flush()
: Discard any bytes that have been written to the client but not yet read.WiFiUDP.stop()
: Disconnect from the server. Release any resource being used during the UDP session.WiFiUDP.remoteIP()
: Gets the IP address of the remote connection. This function must be called afterWiFiUDP.parsePacket()
.WiFiUDP.remotePort()
: Gets the port of the remote UDP connection. This function must be called afterWiFiUDP.parsePacket()
.
Reference
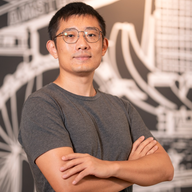